Example usage 1
## [1] 17.67329861 0.09061650 0.12501307 4.46394854 0.03302592 NaN
## [7] 0.08655094 0.04391711 Inf 0.04878161 0.90238403 0.02175046
## [1] "Best distribution based on KL divergence: weibull"
## [1] "Time taken: 0.385"
simulated_data <- simulate_function(n) # Generate 100 samples from the best-fit distribution
par(mfrow = c(1, 2))
hist(vector, main = "Original Data", xlab = "Value", ylab = "Frequency")
hist(simulated_data, main = "Simulated Data", xlab = "Value", ylab = "Frequency")
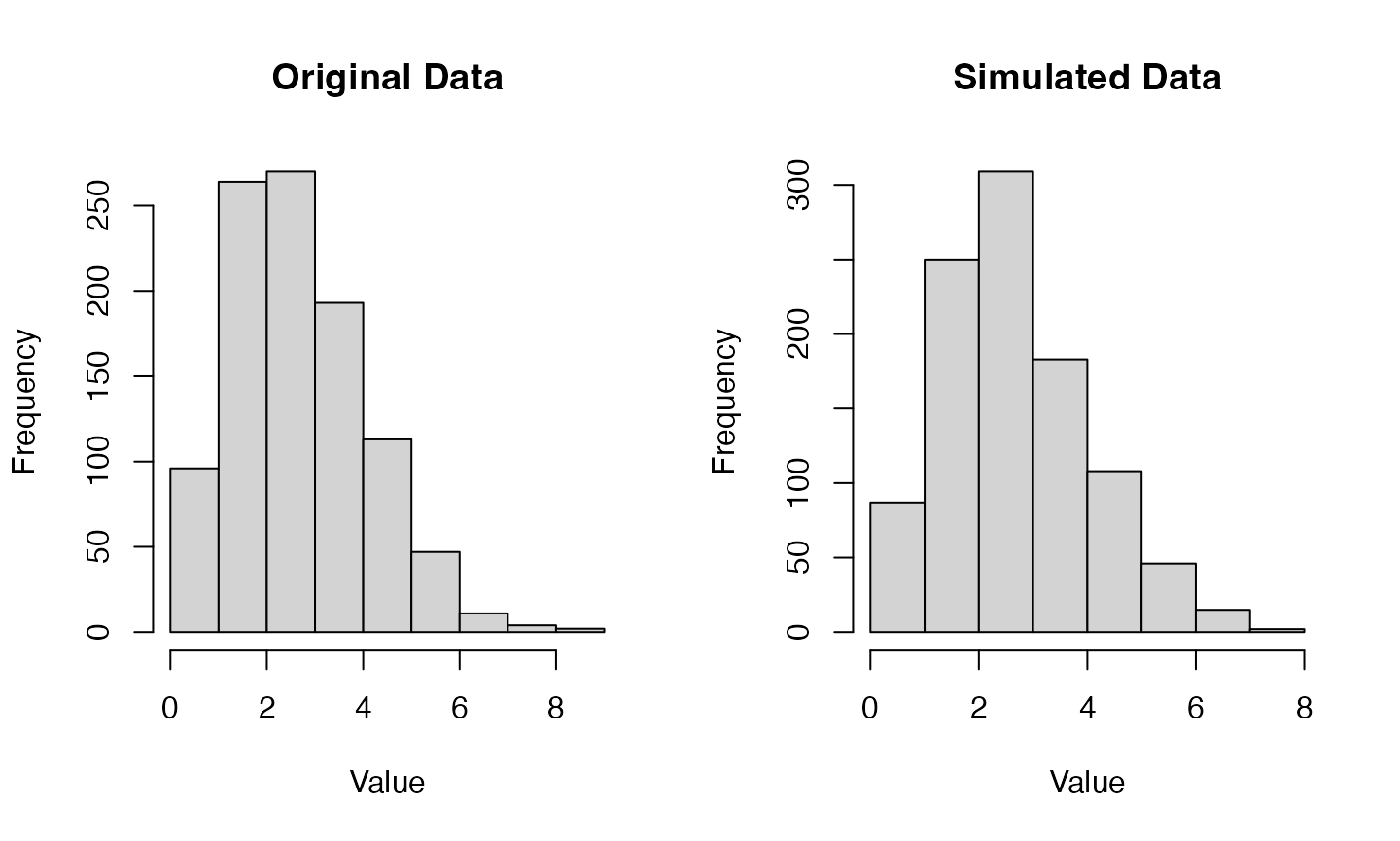
Example usage 2
## [1] 12.56009975 0.06457125 9.61265383 9.60274199 9.57615179 NaN
## [7] Inf 0.01611113 Inf 0.01283163 0.15734994 Inf
## [1] "Best distribution based on KL divergence: normal"
## [1] "Time taken: 0.207"
simulated_data <- simulate_function(n) # Generate 100 samples from the best-fit distribution
par(mfrow = c(1, 2))
hist(vector, main = "Original Data", xlab = "Value", ylab = "Frequency")
hist(simulated_data, main = "Simulated Data", xlab = "Value", ylab = "Frequency")
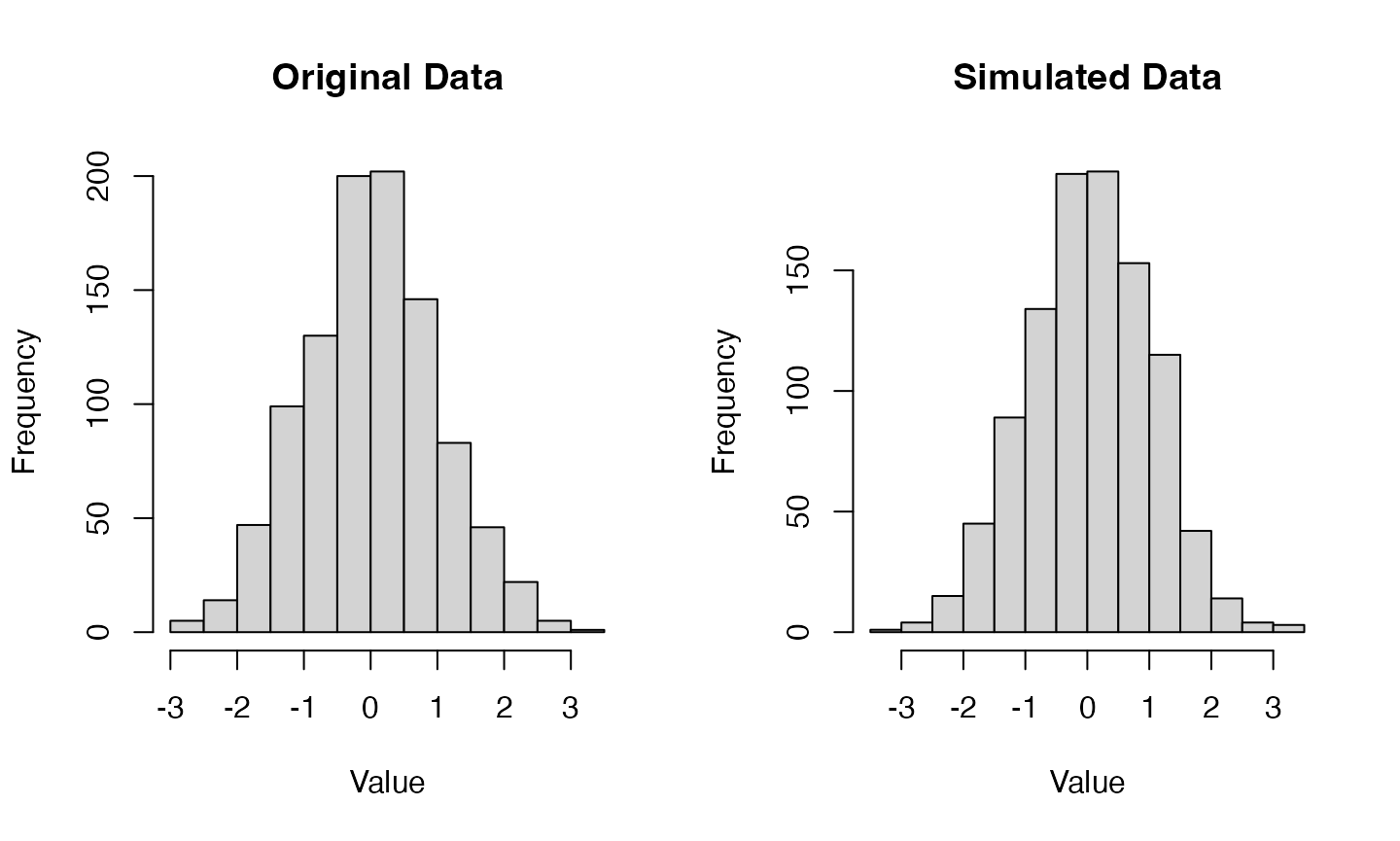
Example usage 3
## [1] 10.15776279 0.03482640 0.04704209 0.70874223 0.05123187 NaN
## [7] 0.01779376 0.25579394 Inf 0.41587181 0.04209187 0.04123623
## [1] "Best distribution based on KL divergence: log-normal"
## [1] "Time taken: 0.377"
simulated_data <- simulate_function(n) # Generate 100 samples from the best-fit distribution
par(mfrow = c(1, 2))
hist(vector, main = "Original Data", xlab = "Value", ylab = "Frequency")
hist(simulated_data, main = "Simulated Data", xlab = "Value", ylab = "Frequency")
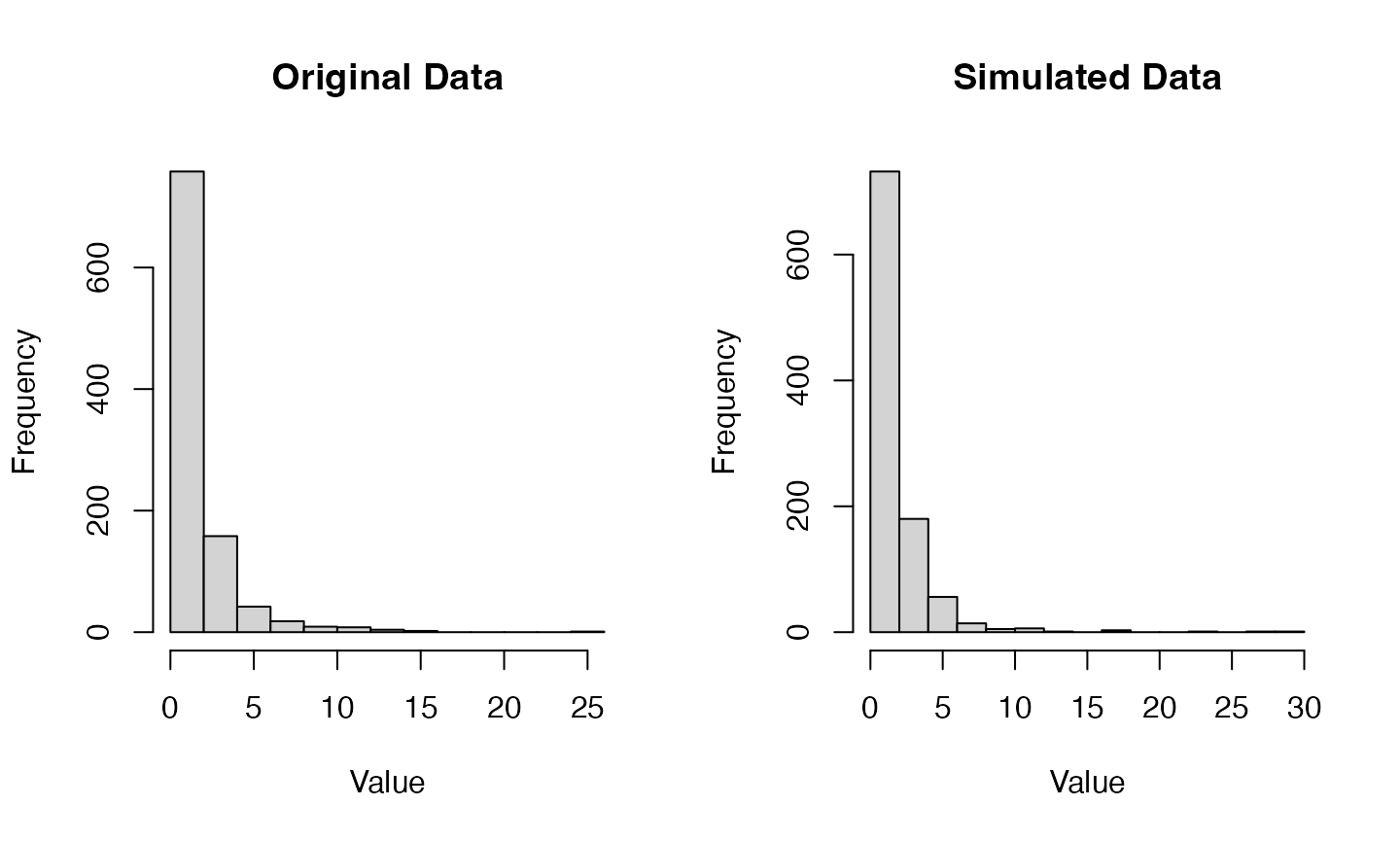
Example usage 4
## [1] 0.003348187 0.076889442 0.317792831 0.192600862 0.037330018 NaN
## [7] 0.107711307 0.025396848 Inf 0.016032465 Inf 0.011174278
## [1] "Best distribution based on KL divergence: beta"
## [1] "Time taken: 0.423999999999999"
simulated_data <- simulate_function(n) # Generate 100 samples from the best-fit distribution
par(mfrow = c(1, 2))
hist(vector, main = "Original Data", xlab = "Value", ylab = "Frequency")
hist(simulated_data, main = "Simulated Data", xlab = "Value", ylab = "Frequency")
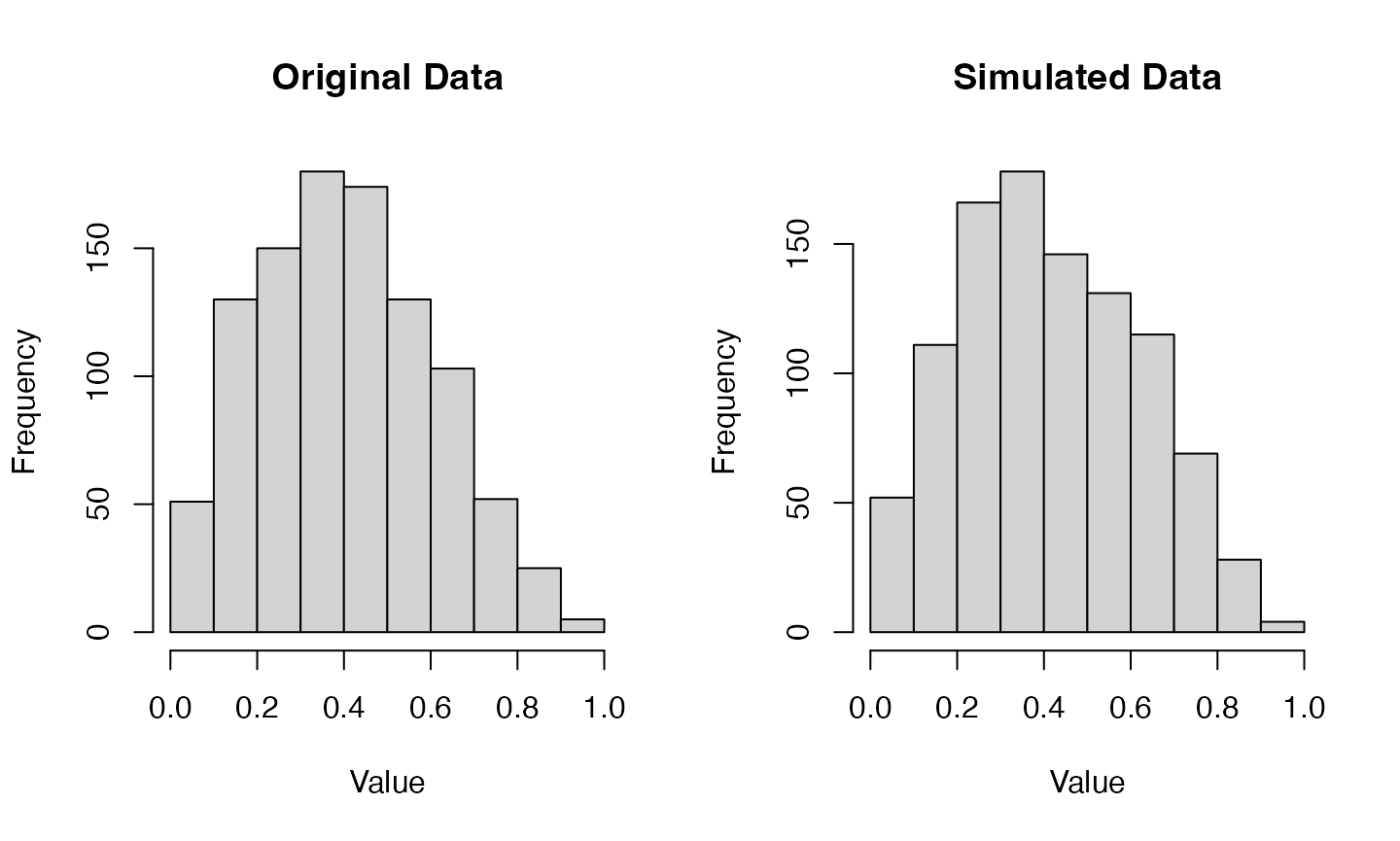